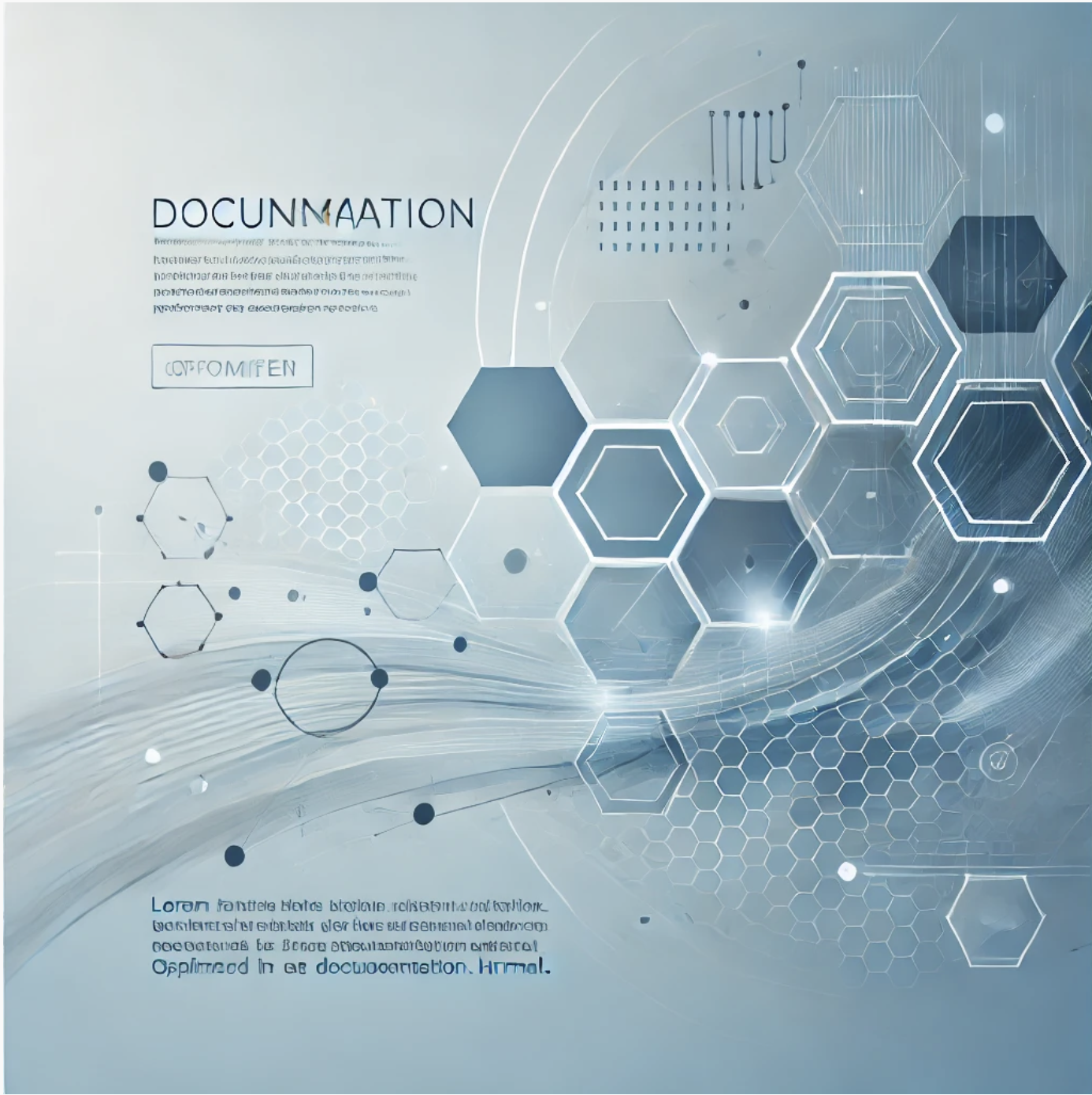
Introduction
The Crypto Insurance Platform is a decentralized protocol for parametric insurance products built on blockchain technology.
Our platform enables the creation, distribution, and settlement of insurance policies in a transparent, automated, and trustless manner. By leveraging smart contracts and oracles, we eliminate intermediaries while providing reliable coverage for various risks.
Key Features
- Fully decentralized insurance platform
- Parametric insurance products with automated claims processing
- Transparent risk pools and premiums
- Staking mechanism for capital provision
- Oracle integration for reliable data feeds
Insurance On The Blockchain
Traditional insurance relies on centralized entities to assess claims, manage risk pools, and determine payouts. This process is often slow, lacks transparency, and introduces trust issues. Our platform addresses these challenges by:
Automation
Smart contracts automatically execute claims based on predefined parameters without human intervention.
Transparency
All transactions, policies, and claims are recorded on the blockchain, providing full transparency.
Efficiency
Automated processes reduce overhead costs and allow for faster policy creation and claim settlements.
Accessibility
Global accessibility allows anyone with a crypto wallet to purchase insurance or provide capital.
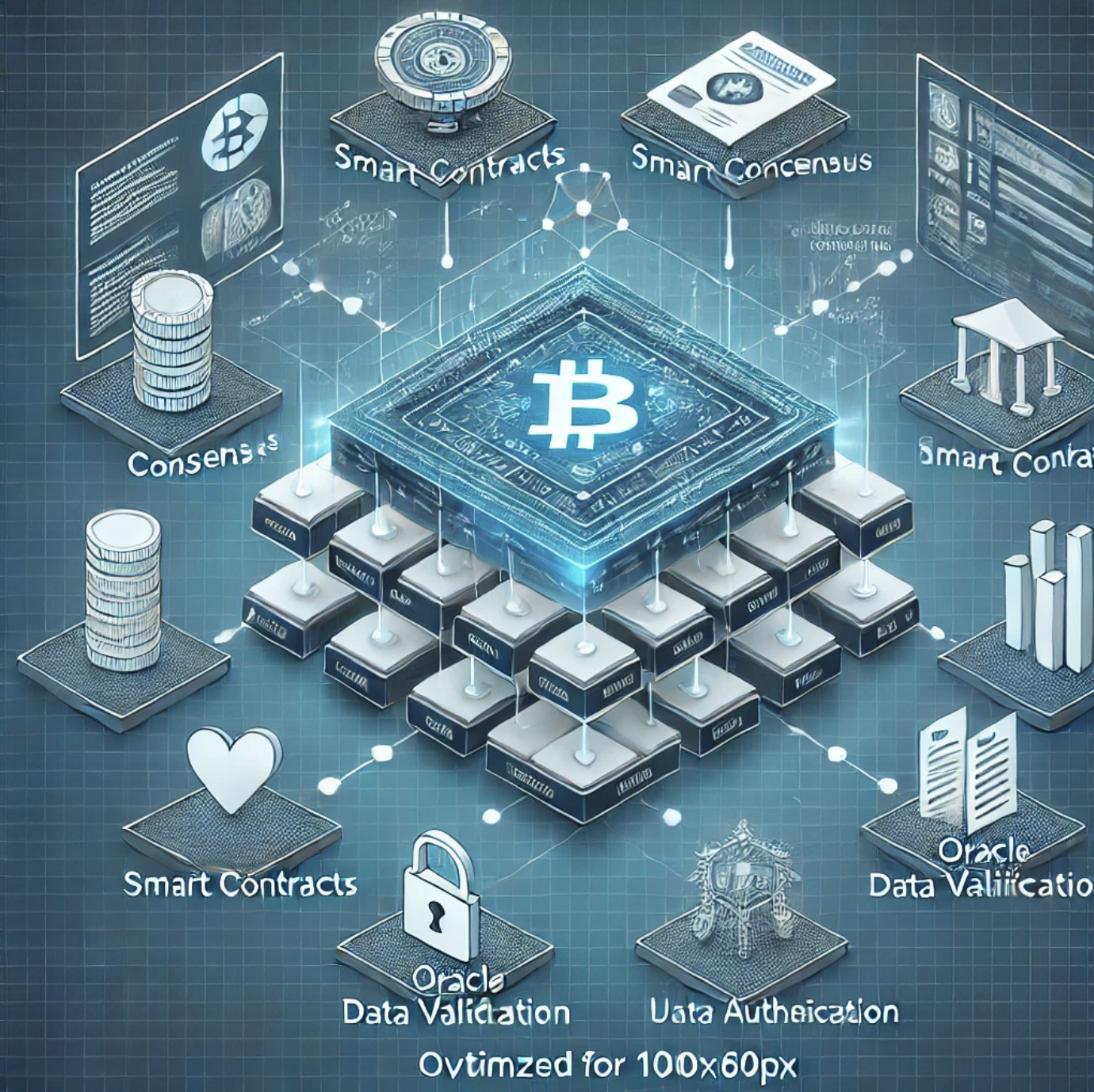
Platform Architecture
The Crypto Insurance Platform consists of several core components that work together to provide a complete decentralized insurance solution.
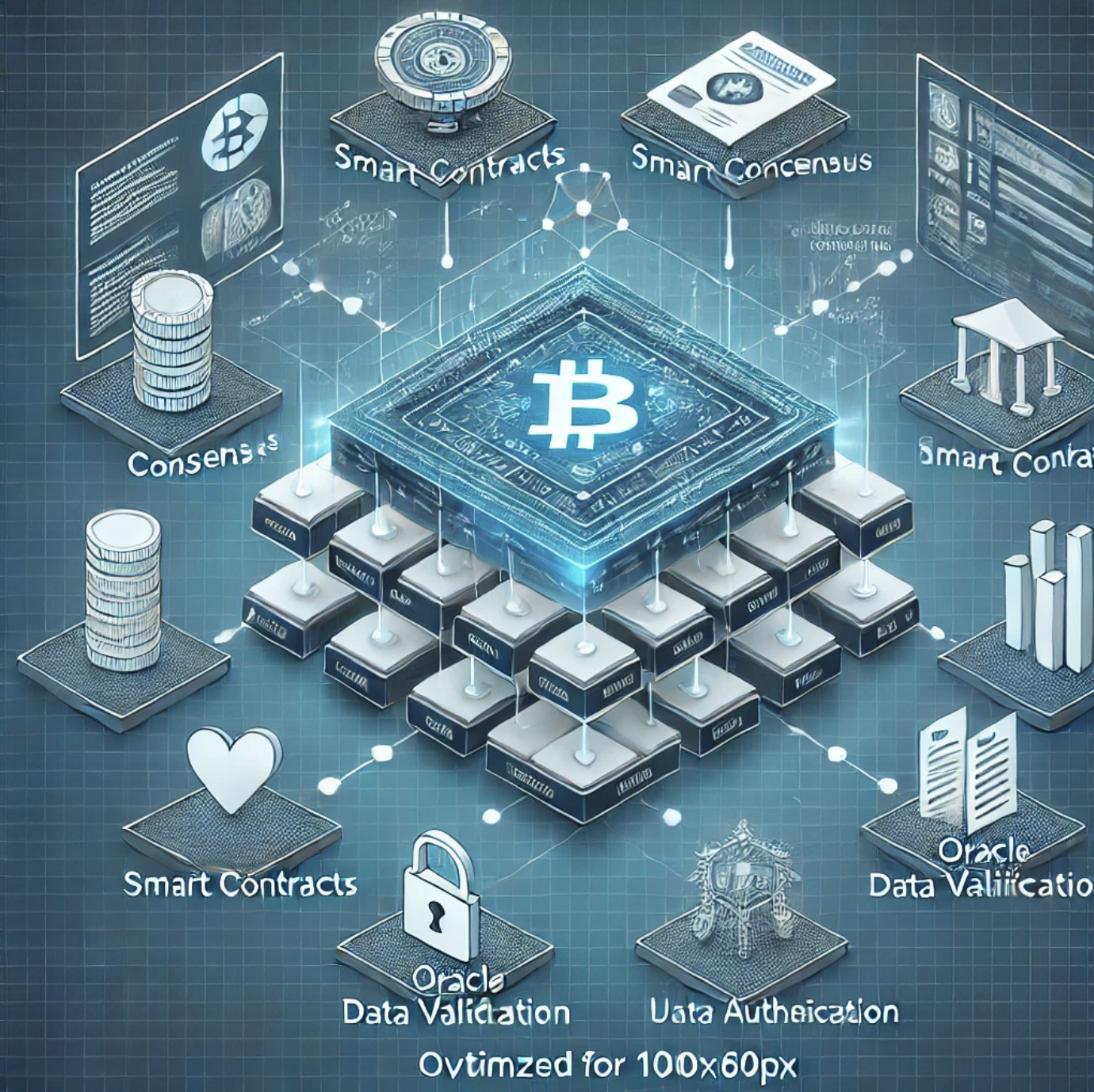
High-level architecture of the Crypto Insurance Platform
Core Components
Insurance Registry
Central registry that maintains a list of all supported insurance products, stake providers, and policy holders.
// Insurance Registry Interface
interface IInsuranceRegistry {
function registerProduct(address productAddress) external;
function registerStakeProvider(address provider) external;
function getProductList() external view returns(address[] memory);
function isValidProduct(address productAddress) external view returns(bool);
}
Policy Manager
Handles the creation, management, and settlement of insurance policies across different product types.
Staking Pool
Manages capital provided by stakers that backs insurance policies and distributes rewards.
Oracle Integration
Connects to external data sources to verify insurance events and trigger automated claims.
Token System
Includes platform tokens used for governance, staking rewards, and premium payments.
Interaction Flow
-
1
Policy Creation
Users select an insurance product, specify coverage parameters, and pay premiums.
-
2
Premium Allocation
Premiums are allocated to staking pools that back the specific insurance product.
-
3
Event Monitoring
Oracle networks monitor external data sources for insured events (e.g., flight delays, stablecoin depeg).
-
4
Claim Verification
Smart contracts verify claim conditions based on oracle data.
-
5
Payout Execution
Upon verification, policy contracts automatically execute payouts to policyholders.
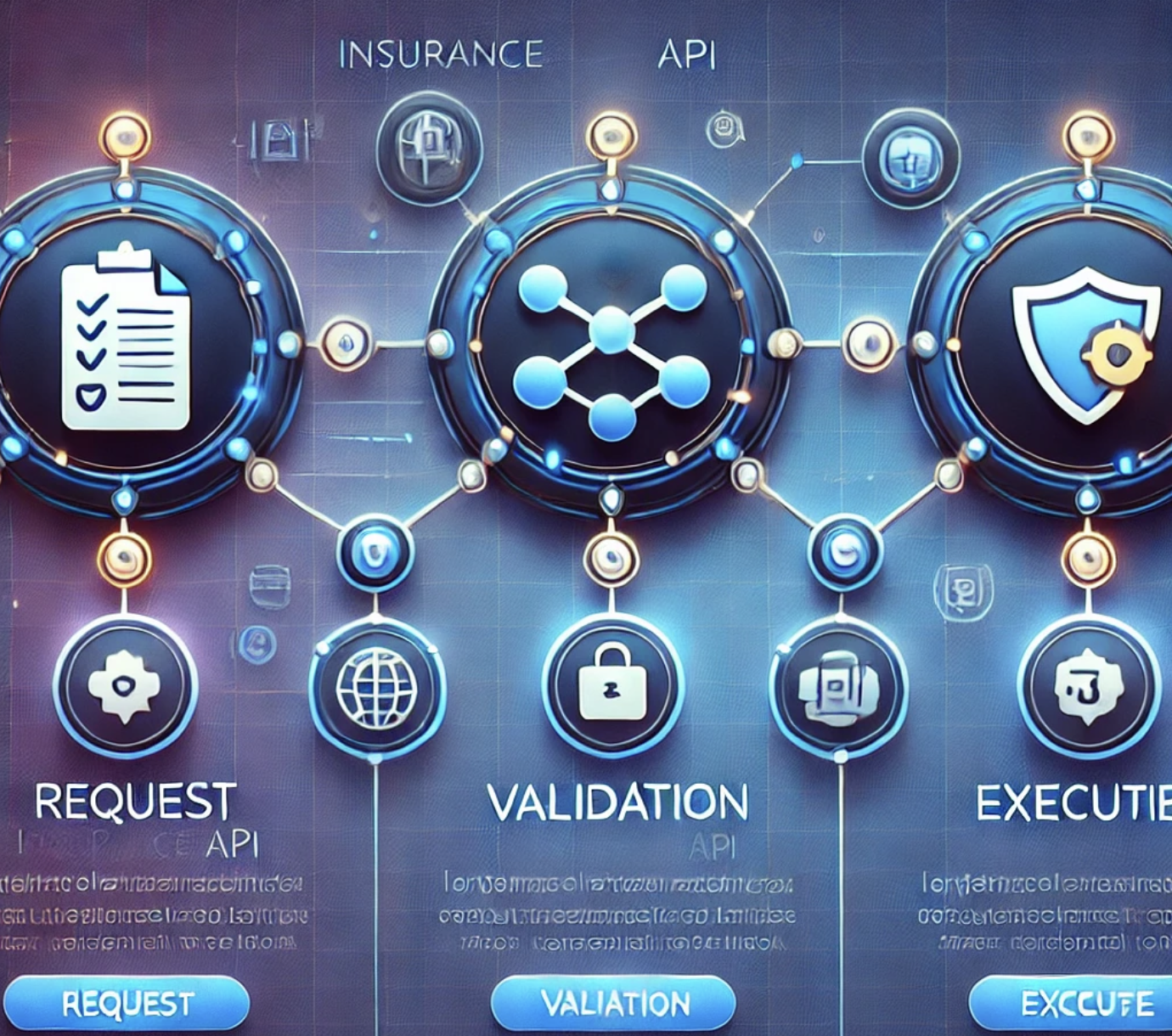
Quick Start Guide
Get started with the Crypto Insurance Platform in just a few steps:
Getting Started as a User
Connect Your Wallet
Visit the platform website and connect your Ethereum wallet (MetaMask, WalletConnect, etc.).
Connect Wallet GuideSelect an Insurance Product
Browse available insurance products and select one that matches your needs.
Customize Your Policy
Set parameters such as coverage amount, duration, and specific conditions.
Pay Premium
Pay the calculated premium in supported cryptocurrencies to activate your policy.
Manage Your Policy
Track your active policies and claims through the user dashboard.
Getting Started as a Staker
Connect Your Wallet
Visit the staking section and connect your wallet.
Select a Staking Pool
Choose a staking pool based on the risk profile and expected returns.
Deposit Funds
Deposit your crypto assets into the selected pool and set your preferred lock period.
Monitor Rewards
Track your staking rewards and pool performance through the staking dashboard.
Withdraw or Reinvest
Choose to withdraw your funds after the lock period or reinvest to compound returns.
Getting Started as a Developer
Clone the Repository
git clone https://github.com/crypto-insurance/platform.git
cd platform
npm install
Set Up Local Environment
# Create .env file with your configuration
cp .env.example .env
# Edit .env with your settings
npm run setup
Run Local Development Node
npm run node
Deploy Smart Contracts
npm run deploy:local
Integrate with Your Application
Use our SDK to integrate insurance products into your application.
SDK DocumentationFlight Delay Insurance
Flight delay insurance provides compensation to travelers when their flights are delayed beyond a specified threshold.
Product Parameters
Parameter | Description | Default |
---|---|---|
Flight Number | IATA/ICAO flight identifier | Required |
Departure Date | Scheduled departure date | Required |
Delay Threshold | Minimum delay time (minutes) to trigger payout | 60 minutes |
Coverage Amount | Maximum payout amount | Configurable |
Premium Rate | Percentage of coverage amount | 5-15% |
Oracle Integration
Flight delay insurance relies on flight status data from multiple sources:
- FlightStats API
- Chainlink Data Feeds
- Airport authority data feeds
The oracle network aggregates data from these sources to ensure accuracy and reliability. A minimum of 3 out of 5 data sources must confirm a delay before triggering a payout.
Policy Lifecycle
Policy Creation
User purchases policy by specifying flight details and coverage amount
Flight Monitoring
Oracle network tracks flight status in real-time
Delay Detection
System detects if the flight is delayed beyond threshold
Automatic Payout
Smart contract executes payout to user's wallet
Policy Closure
Policy is marked as claimed and archived
Integration Example
// Creating a flight delay insurance policy
const { FlightInsurance } = require('@crypto-insurance/sdk');
// Initialize SDK with your API key
const flightInsurance = new FlightInsurance({
apiKey: 'YOUR_API_KEY',
network: 'mainnet'
});
// Create a policy
async function createPolicy() {
const policy = await flightInsurance.createPolicy({
flightNumber: 'UA123',
departureDate: '2025-03-15',
departureTime: '14:30',
delayThreshold: 90, // minutes
coverageAmount: 500, // in USD
policyholderAddress: '0x742d35Cc6634C0532925a3b844Bc454e4438f44e'
});
console.log(`Policy created with ID: ${policy.id}`);
return policy;
}
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.0;
import "@crypto-insurance/contracts/FlightInsurancePolicy.sol";
import "@chainlink/contracts/src/v0.8/ChainlinkClient.sol";
contract FlightDelayPolicy is FlightInsurancePolicy, ChainlinkClient {
string public flightNumber;
uint256 public departureDate;
uint256 public delayThreshold;
uint256 public coverageAmount;
address public policyholder;
enum PolicyStatus { Active, PaidOut, Expired }
PolicyStatus public status;
event PolicyCreated(string flightNumber, uint256 departureDate);
event PolicyPaidOut(uint256 amount);
constructor(
string memory _flightNumber,
uint256 _departureDate,
uint256 _delayThreshold,
uint256 _coverageAmount,
address _policyholder
) {
flightNumber = _flightNumber;
departureDate = _departureDate;
delayThreshold = _delayThreshold;
coverageAmount = _coverageAmount;
policyholder = _policyholder;
status = PolicyStatus.Active;
emit PolicyCreated(flightNumber, departureDate);
}
}
function processClaim(uint256 delayDuration) external onlyOracle {
require(status == PolicyStatus.Active, "Policy not active");
if (delayDuration >= delayThreshold) {
status = PolicyStatus.PaidOut;
// Transfer coverage amount to policyholder
(bool success, ) = policyholder.call{value: coverageAmount}("");
require(success, "Transfer failed");
emit PolicyPaidOut(coverageAmount);
}
}
}
}
}
Event Cancellation Insurance
Event cancellation insurance protects ticket holders against financial loss when events are cancelled, postponed, or relocated.
Product Parameters
Parameter | Description | Default |
---|---|---|
Event ID | Unique identifier for the event | Required |
Event Date | Scheduled date of the event | Required |
Event Type | Category of the event (concert, sports, conference, etc.) | Required |
Ticket Value | Purchase price of the ticket(s) | Required |
Coverage Amount | Maximum payout amount (typically equal to ticket value) | Equal to ticket value |
Premium Rate | Percentage of coverage amount | 8-20% |
Covered Scenarios
Event cancellation insurance covers the following scenarios:
- Cancellation: Event is completely cancelled by the organizer
- Postponement: Event is rescheduled to a later date
- Venue Change: Event is relocated to a different venue
- Performer No-Show: Main performer(s) cannot appear
The policy does not cover voluntary non-attendance or inability to attend due to personal circumstances.
Oracle Integration
Event cancellation verification relies on data from multiple authoritative sources:
- Official event organizer announcements
- Venue information systems
- Ticketing platform APIs
- Media and news verification services
The oracle network requires confirmation from at least two independent sources to verify an event cancellation or significant change before processing a claim.
Integration Example
// Creating an event cancellation insurance policy
const { EventInsurance } = require('@crypto-insurance/sdk');
// Initialize SDK with your API key
const eventInsurance = new EventInsurance({
apiKey: 'YOUR_API_KEY',
network: 'mainnet'
});
// Create a policy
async function createEventPolicy() {
const policy = await eventInsurance.createPolicy({
eventId: 'EV-2025-06-123',
eventName: 'Summer Music Festival 2025',
eventDate: '2025-06-15',
eventType: 'concert',
ticketValue: 250, // in USD
coverageAmount: 250, // in USD
policyholderAddress: '0x742d35Cc6634C0532925a3b844Bc454e4438f44e'
});
console.log(`Event policy created with ID: ${policy.id}`);
return policy;
}
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.0;
import "@crypto-insurance/contracts/EventInsurancePolicy.sol";
import "@chainlink/contracts/src/v0.8/ChainlinkClient.sol";
contract EventCancellationPolicy is EventInsurancePolicy, ChainlinkClient {
string public eventId;
string public eventName;
uint256 public eventDate;
string public eventType;
uint256 public ticketValue;
uint256 public coverageAmount;
address public policyholder;
enum PolicyStatus { Active, PaidOut, Expired }
enum CancellationReason { Cancelled, Postponed, VenueChanged, PerformerNoShow }
PolicyStatus public status;
event PolicyCreated(string eventId, string eventName, uint256 eventDate);
event PolicyPaidOut(uint256 amount, CancellationReason reason);
constructor(
string memory _eventId,
string memory _eventName,
uint256 _eventDate,
string memory _eventType,
uint256 _ticketValue,
address _policyholder
) {
eventId = _eventId;
eventName = _eventName;
eventDate = _eventDate;
eventType = _eventType;
ticketValue = _ticketValue;
coverageAmount = _ticketValue;
policyholder = _policyholder;
status = PolicyStatus.Active;
emit PolicyCreated(eventId, eventName, eventDate);
}
}
function processClaim(bytes calldata oracleData) external onlyOracle {
require(status == PolicyStatus.Active, "Policy not active");
// Decode oracle data
(bool eventCancelled, CancellationReason reason) = abi.decode(
oracleData,
(bool, CancellationReason)
);
if (eventCancelled) {
status = PolicyStatus.PaidOut;
// Transfer coverage amount to policyholder
(bool success, ) = policyholder.call{value: coverageAmount}("");
require(success, "Transfer failed");
emit PolicyPaidOut(coverageAmount, reason);
}
}
}
}
}
Stablecoin Depeg Insurance
Stablecoin depeg insurance protects holders against significant price deviations from the pegged value of stablecoins.
Product Parameters
Parameter | Description | Default |
---|---|---|
Stablecoin | The stablecoin to insure (USDC, USDT, DAI, etc.) | Required |
Coverage Amount | Amount of stablecoin to be insured | Required |
Depeg Threshold | Price deviation that triggers a payout (e.g., 0.95 or 0.90) | 0.95 USD |
Duration | Length of coverage period | 30 days |
Premium Rate | Percentage of coverage amount | 1-5% |
Observation Period | Time period price must remain below threshold | 1 hour |
Oracle Integration
Stablecoin price data is collected from multiple sources to ensure accuracy:
- Centralized exchanges (Coinbase, Binance, Kraken)
- Decentralized exchanges (Uniswap, Curve, SushiSwap)
- Chainlink Price Feeds
- Band Protocol
- API3
The oracle system calculates a volume-weighted average price (VWAP) across all sources and requires the price to remain below the depeg threshold for the entire observation period before triggering a payout.
Payout Calculation
The payout amount is calculated based on the severity of the depeg event:
// Pseudocode for payout calculation
function calculatePayout(coverageAmount, depegThreshold, actualPrice) {
if (actualPrice >= depegThreshold) {
return 0; // No payout if price is above threshold
}
// Calculate the percentage deviation from $1
const deviation = 1 - actualPrice;
// Calculate payout based on deviation percentage
const payoutPercentage = Math.min(1, deviation * 2); // Cap at 100%
return coverageAmount * payoutPercentage;
}
For example, if a user insures 10,000 USDC and the price drops to $0.85:
- Deviation: 1 - 0.85 = 0.15 (15%)
- Payout percentage: 0.15 * 2 = 0.3 (30%)
- Payout: 10,000 * 0.3 = 3,000 USDC
Integration Example
// Creating a stablecoin depeg insurance policy
const { StablecoinInsurance } = require('@crypto-insurance/sdk');
// Initialize SDK with your API key
const stablecoinInsurance = new StablecoinInsurance({
apiKey: 'YOUR_API_KEY',
network: 'mainnet'
});
// Create a policy
async function createStablecoinPolicy() {
const policy = await stablecoinInsurance.createPolicy({
stablecoin: 'USDC',
coverageAmount: 10000, // 10,000 USDC
depegThreshold: 0.95, // 95 cents
duration: 30, // days
observationPeriod: 60, // minutes
policyholderAddress: '0x742d35Cc6634C0532925a3b844Bc454e4438f44e'
});
console.log(`Stablecoin policy created with ID: ${policy.id}`);
return policy;
}
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.0;
import "@crypto-insurance/contracts/StablecoinInsurancePolicy.sol";
import "@chainlink/contracts/src/v0.8/ChainlinkClient.sol";
contract StablecoinDepegPolicy is StablecoinInsurancePolicy, ChainlinkClient {
string public stablecoin;
uint256 public coverageAmount;
uint256 public depegThreshold; // Stored as price * 100 (e.g., 95 for $0.95)
uint256 public startTime;
uint256 public endTime;
uint256 public observationPeriod; // in minutes
address public policyholder;
enum PolicyStatus { Active, PaidOut, Expired }
PolicyStatus public status;
event PolicyCreated(string stablecoin, uint256 coverageAmount, uint256 depegThreshold);
event PolicyPaidOut(uint256 amount, uint256 actualPrice);
constructor(
string memory _stablecoin,
uint256 _coverageAmount,
uint256 _depegThreshold,
uint256 _duration,
uint256 _observationPeriod,
address _policyholder
) {
stablecoin = _stablecoin;
coverageAmount = _coverageAmount;
depegThreshold = _depegThreshold;
startTime = block.timestamp;
endTime = block.timestamp + (_duration * 1 days);
observationPeriod = _observationPeriod * 1 minutes;
policyholder = _policyholder;
status = PolicyStatus.Active;
emit PolicyCreated(stablecoin, coverageAmount, depegThreshold);
}
}
function processClaim(bytes calldata oracleData) external onlyOracle {
require(status == PolicyStatus.Active, "Policy not active");
require(block.timestamp <= endTime, "Policy expired");
// Decode oracle data
(uint256 actualPrice, bool belowThresholdForPeriod) = abi.decode(
oracleData,
(uint256, bool)
);
if (actualPrice < depegThreshold && belowThresholdForPeriod) {
status = PolicyStatus.PaidOut;
// Calculate payout amount based on severity
uint256 deviation = 100 - actualPrice;
uint256 payoutPercentage = (deviation * 2 > 100) ? 100 : deviation * 2;
uint256 payoutAmount = (coverageAmount * payoutPercentage) / 100;
// Transfer payout to policyholder
(bool success, ) = policyholder.call{value: payoutAmount}("");
require(success, "Transfer failed");
emit PolicyPaidOut(payoutAmount, actualPrice);
}
}
}
}
}
Weather Impact Insurance
Weather impact insurance provides protection against adverse weather conditions that may affect business operations, events, or agricultural yields.
Product Parameters
Parameter | Description | Default |
---|---|---|
Location | Geographic coordinates or location ID | Required |
Weather Parameter | Specific condition (temperature, rainfall, wind, etc.) | Required |
Threshold | Trigger level for the chosen parameter | Required |
Coverage Period | Start and end dates for coverage | Required |
Coverage Amount | Maximum payout in case of trigger event | Required |
Premium Rate | Percentage of coverage amount | 3-15% |
Weather Parameters
The protocol supports a wide range of weather parameters:
- Temperature: Maximum, minimum, or average (°C/°F)
- Precipitation: Rainfall or snowfall amount (mm/inches)
- Wind: Speed, direction, or gust strength
- Sunshine: Hours of sunlight
- Extreme Events: Hurricanes, floods, droughts
Oracle Integration
Weather data is sourced from multiple professional meteorological services:
- National Weather Service
- AccuWeather
- Weather Underground
- The Weather Company
- Local weather stations network
Data from at least three independent sources is aggregated and verified before triggering a policy payout. For historical data verification, the system also uses satellite imagery and ground-based sensor networks.
Use Cases
Agriculture
Protection against drought, excessive rainfall, or unseasonal frost affecting crop yields
Outdoor Events
Coverage for concerts, festivals, or sports events impacted by adverse weather
Seasonal Businesses
Protection for businesses dependent on specific weather conditions (ski resorts, beach venues)
Construction
Coverage against project delays caused by adverse weather conditions
Integration Example
// Creating a weather impact insurance policy
const { WeatherInsurance } = require('@crypto-insurance/sdk');
// Initialize SDK with your API key
const weatherInsurance = new WeatherInsurance({
apiKey: 'YOUR_API_KEY',
network: 'mainnet'
});
// Create a policy
async function createWeatherPolicy() {
const policy = await weatherInsurance.createPolicy({
location: {
latitude: 40.7128,
longitude: -74.0060,
locationName: 'New York, NY'
}
},
weatherParameter: 'RAINFALL',
thresholdType: 'EXCEEDS',
thresholdValue: 25, // mm of rainfall
coveragePeriod: {
startDate: '2025-07-15',
endDate: '2025-07-18'
}
},
coverageAmount: 5000, // in USD
description: 'Outdoor wedding protection',
policyholderAddress: '0x742d35Cc6634C0532925a3b844Bc454e4438f44e'
});
console.log(`Weather policy created with ID: ${policy.id}`);
return policy;
}
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.0;
import "@crypto-insurance/contracts/WeatherInsurancePolicy.sol";
import "@chainlink/contracts/src/v0.8/ChainlinkClient.sol";
contract WeatherImpactPolicy is WeatherInsurancePolicy, ChainlinkClient {
// Location data
int256 public latitude;
int256 public longitude;
string public locationName;
// Weather parameters
enum WeatherParameterType { TEMPERATURE, RAINFALL, SNOWFALL, WIND, SUNSHINE }
enum ThresholdType { EXCEEDS, FALLS_BELOW, BETWEEN, OUTSIDE_RANGE }
WeatherParameterType public weatherParameter;
ThresholdType public thresholdType;
int256 public thresholdValue;
int256 public secondaryThreshold; // For BETWEEN and OUTSIDE_RANGE types
// Policy details
uint256 public startDate;
uint256 public endDate;
uint256 public coverageAmount;
address public policyholder;
string public description;
enum PolicyStatus { Active, PaidOut, Expired }
PolicyStatus public status;
event PolicyCreated(string locationName, uint256 startDate, uint256 endDate);
event PolicyPaidOut(uint256 amount, int256 actualWeatherValue);
constructor(
int256 _latitude,
int256 _longitude,
string memory _locationName,
uint8 _weatherParameter,
uint8 _thresholdType,
int256 _thresholdValue,
int256 _secondaryThreshold,
uint256 _startDate,
uint256 _endDate,
uint256 _coverageAmount,
address _policyholder,
string memory _description
) {
latitude = _latitude;
longitude = _longitude;
locationName = _locationName;
weatherParameter = WeatherParameterType(_weatherParameter);
thresholdType = ThresholdType(_thresholdType);
thresholdValue = _thresholdValue;
secondaryThreshold = _secondaryThreshold;
startDate = _startDate;
endDate = _endDate;
coverageAmount = _coverageAmount;
policyholder = _policyholder;
description = _description;
status = PolicyStatus.Active;
emit PolicyCreated(locationName, startDate, endDate);
}
}
function processClaim(bytes calldata oracleData) external onlyOracle {
require(status == PolicyStatus.Active, "Policy not active");
require(block.timestamp <= endDate, "Policy expired");
// Decode oracle data
(int256 actualWeatherValue, bool conditionMet) = abi.decode(
oracleData,
(int256, bool)
);
if (conditionMet) {
status = PolicyStatus.PaidOut;
// Transfer coverage amount to policyholder
(bool success, ) = policyholder.call{value: coverageAmount}("");
require(success, "Transfer failed");
emit PolicyPaidOut(coverageAmount, actualWeatherValue);
}
}
}
}
function isThresholdMet(int256 value) internal view returns (bool) {
if (thresholdType == ThresholdType.EXCEEDS) {
return value > thresholdValue;
}
} else if (thresholdType == ThresholdType.FALLS_BELOW) {
return value < thresholdValue;
}
} else if (thresholdType == ThresholdType.BETWEEN) {
return value >= thresholdValue && value <= secondaryThreshold;
}
} else if (thresholdType == ThresholdType.OUTSIDE_RANGE) {
return value < thresholdValue || value > secondaryThreshold;
}
}
return false;
}
}
}
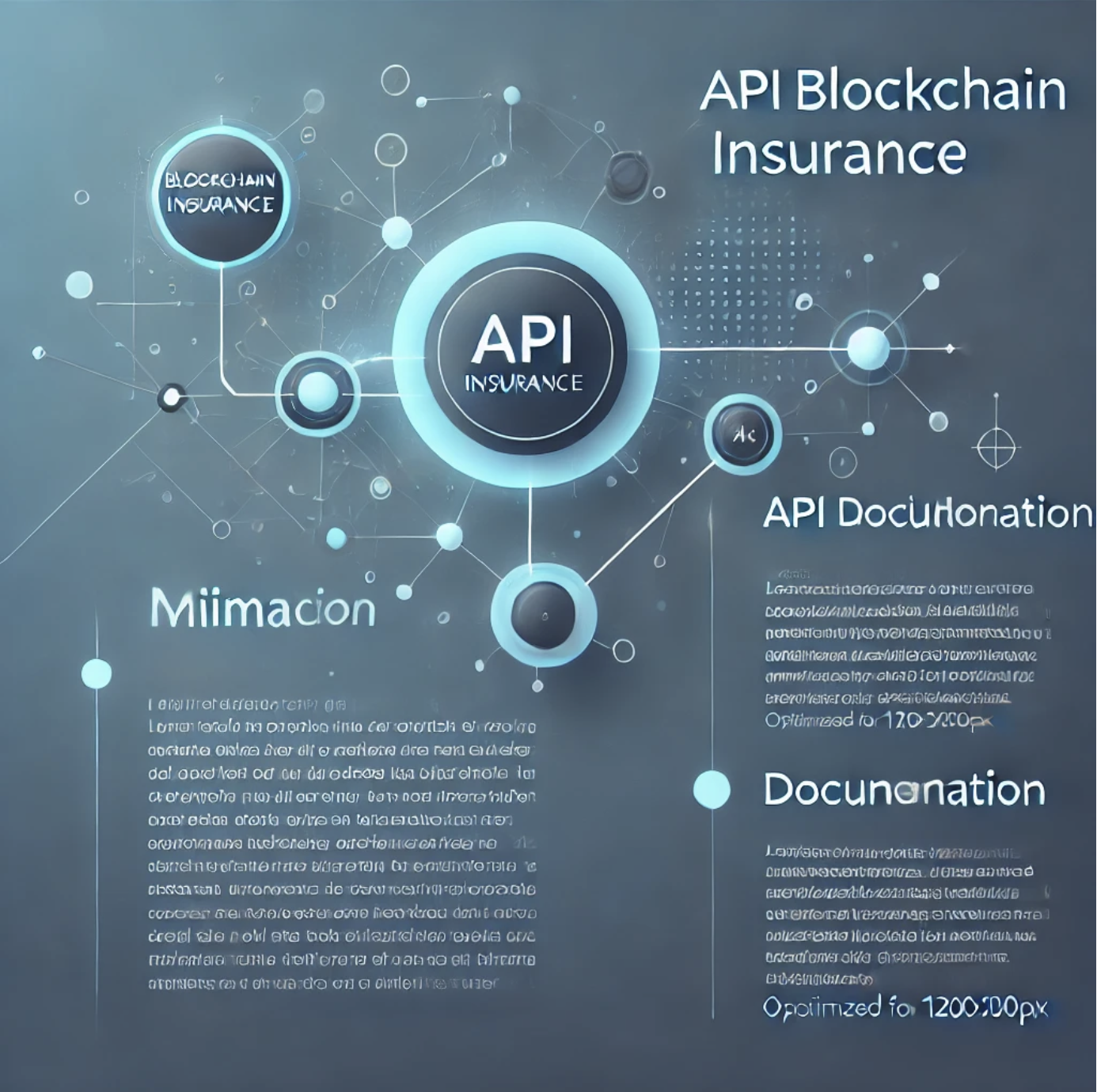
API Reference
The DefiLean API allows developers to interact with our insurance products and services programmatically.
Our RESTful API provides endpoints for creating insurance policies, querying policy status, managing claims, and integrating with the staking system. This enables third-party applications to offer our insurance products directly within their platforms.
API Access Requirements
- API keys for authentication
- SSL encryption for all API requests
- Rate limiting applies to prevent abuse
- Webhook setup for real-time event notifications
API Versioning
The API uses versioning to ensure backward compatibility as we add new features. The current version is v1, which is specified in the URL path.
https://api.defilean.io/v1/policies
We commit to maintaining backward compatibility within a major version. Breaking changes will only be introduced in new major versions, and we'll provide migration guides and deprecation notices well in advance.
API Authentication
Authentication for the DefiLean API uses API keys to identify your application and JWT tokens for user-specific operations.
API Keys
API keys are used to authenticate your application. You can generate API keys in the Developer Dashboard.
// Example API request with API key
fetch('https://api.defilean.io/v1/policies', {
method: 'GET',
headers: {
'Content-Type': 'application/json',
'X-API-Key': 'your_api_key_here'
}
})
API Key Security
Never expose your API keys in client-side code. Always keep them secure on your server. If you suspect an API key has been compromised, you should immediately rotate it in the Developer Dashboard.
User Authentication
For operations that require user authentication, you'll need to use JSON Web Tokens (JWT). These tokens can be obtained by implementing our authentication flow.
// Example authenticated user request
fetch('https://api.defilean.io/v1/user/policies', {
method: 'GET',
headers: {
'Content-Type': 'application/json',
'X-API-Key': 'your_api_key_here',
'Authorization': 'Bearer your_jwt_token_here'
}
})
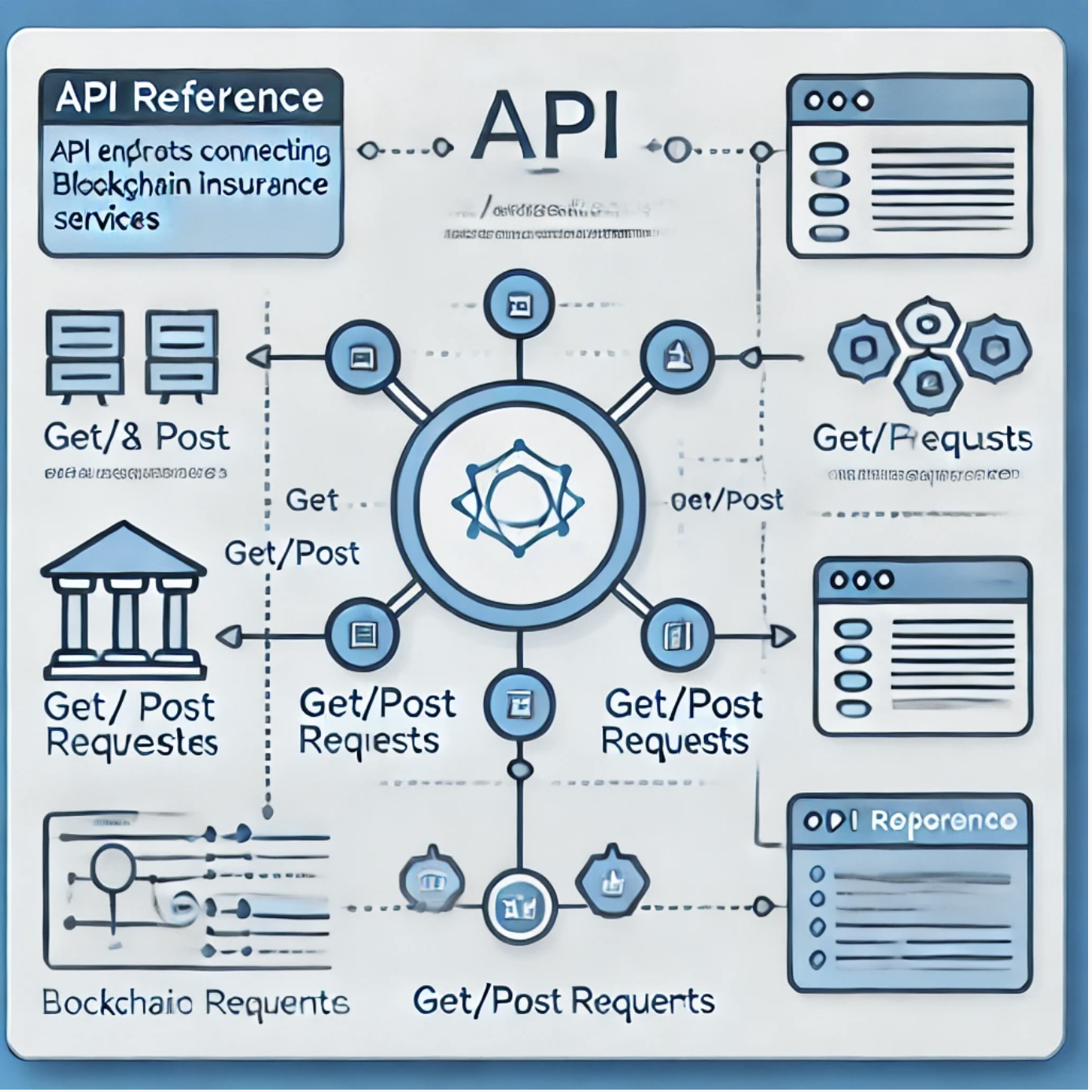
API Endpoints
The DefiLean API provides the following key endpoints:
Endpoint | Method | Description |
---|---|---|
/v1/policies |
GET | List all available insurance policies |
/v1/policies/{id} |
GET | Get details of a specific policy |
/v1/policies |
POST | Create a new insurance policy |
/v1/claims |
GET | List all claims associated with authenticated user |
/v1/claims/{id} |
GET | Get details of a specific claim |
/v1/stakes |
GET | List available staking opportunities |
/v1/stakes |
POST | Create a new staking position |
/v1/user/policies |
GET | List policies owned by authenticated user |
/v1/user/stakes |
GET | List staking positions owned by authenticated user |
Request Example
Here's an example of creating a new flight delay insurance policy:
// Creating a flight delay policy
fetch('https://api.defilean.io/v1/policies', {
method: 'POST',
headers: {
'Content-Type': 'application/json',
'X-API-Key': 'your_api_key_here',
'Authorization': 'Bearer user_jwt_token'
},
body: JSON.stringify({
type: 'flight_delay',
parameters: {
flightNumber: 'UA123',
departureDate: '2025-03-15',
departureTime: '14:30',
delayThreshold: 90,
coverageAmount: 500
}
},
premiumCurrency: 'USDC'
})
})
Response Example
{
"status": "success",
"data": {
"policyId": "pol_f8d7a6c5b4e3",
"type": "flight_delay",
"parameters": {
"flightNumber": "UA123",
"departureDate": "2025-03-15",
"departureTime": "14:30",
"delayThreshold": 90,
"coverageAmount": 500
}
},
"premium": {
"amount": 35,
"currency": "USDC"
}
},
"status": "pending_payment",
"paymentUrl": "https://pay.defilean.io/checkout/f8d7a6c5b4e3",
"expiresAt": "2025-03-01T14:30:00Z",
"createdAt": "2025-02-15T10:45:32Z"
}
}
Webhooks
Webhooks allow your application to receive real-time notifications about events in the DefiLean platform, such as policy creation, claim processing, and payouts.
Setting Up Webhooks
You can configure webhook endpoints in the Developer Dashboard. When setting up a webhook, you need to provide:
- The URL where you want to receive webhook events
- The types of events you want to subscribe to
- An optional secret key for signature verification
Event Types
DefiLean supports the following webhook event types:
Policy Events
policy.created, policy.activated, policy.expired
Claim Events
claim.initiated, claim.verified, claim.processed
Payment Events
payment.received, payment.failed, payout.sent
Staking Events
stake.created, stake.rewarded, stake.withdrawn
Webhook Payload
Webhook payloads are sent as JSON objects in the body of the HTTP POST request. Each payload includes:
{
"id": "evt_123456789",
"type": "policy.created",
"created": "2025-02-15T10:45:32Z",
"data": {
// Event-specific data
"policyId": "pol_f8d7a6c5b4e3",
"type": "flight_delay",
"customer": "cus_abcdef123456",
"status": "pending_payment"
}
}
Signature Verification
To verify that webhooks are coming from DefiLean and not an unauthorized party, we include a signature in the X-DefiLean-Signature
header. You should verify this signature using your webhook secret.
// Node.js example for verifying webhook signatures
const crypto = require('crypto');
function verifyWebhookSignature(payload, signature, secret) {
const hmac = crypto.createHmac('sha256', secret);
const expectedSignature = hmac.update(payload).digest('hex');
return crypto.timingSafeEqual(
Buffer.from(signature),
Buffer.from(expectedSignature)
);
}
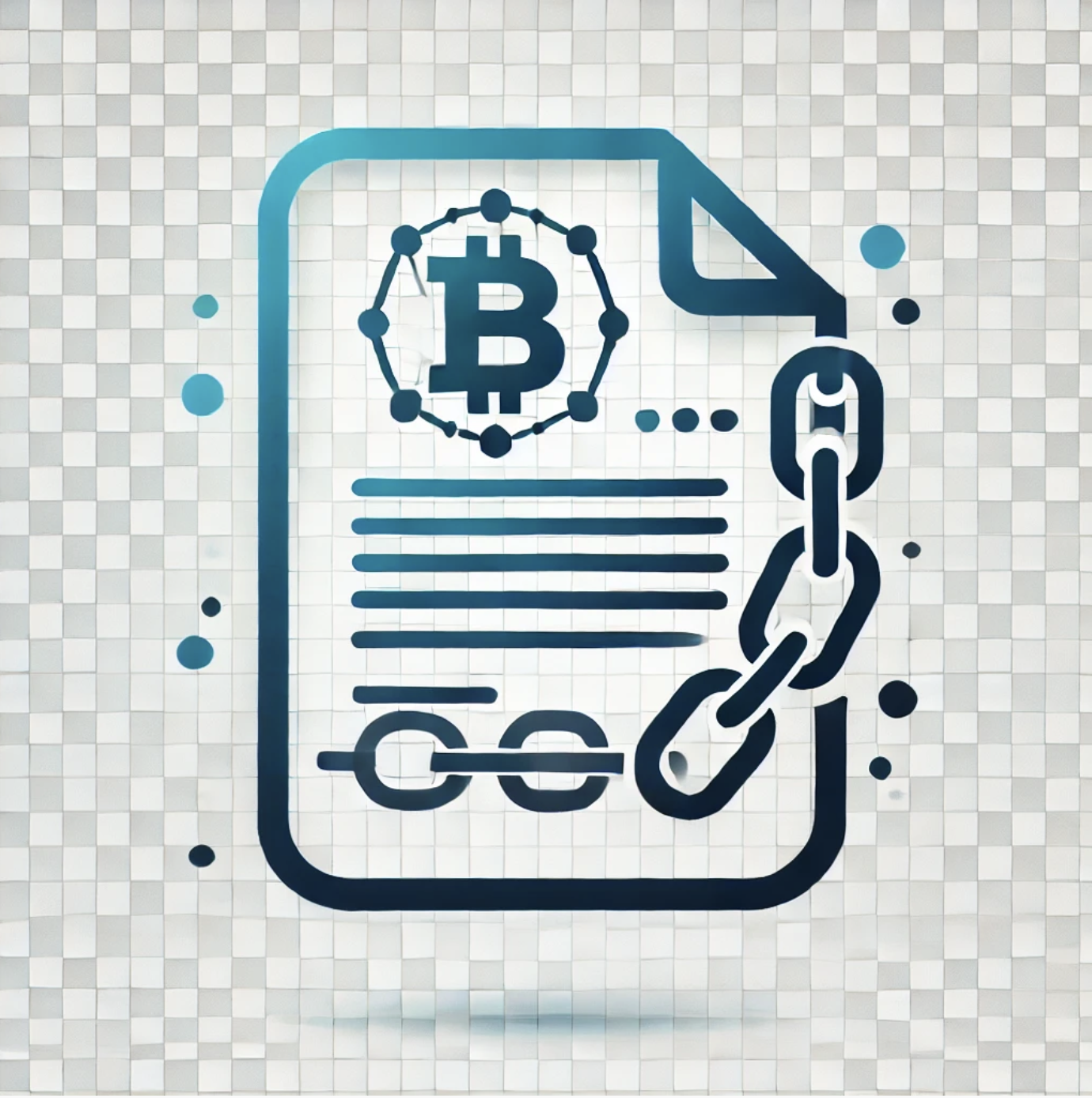
Smart Contracts Overview
DefiLean's infrastructure is built on Ethereum-compatible smart contracts that enable trustless insurance policies and staking.
Our smart contracts are designed with security, transparency, and efficiency in mind. They handle all aspects of the insurance lifecycle, from policy creation to automated claim payouts, while ensuring that funds are properly managed and secured.
Security Measures
- All contracts have undergone thorough security audits by top firms
- Time-locked upgrades with multi-signature governance
- Insurance fund to protect against extreme events
- Circuit breakers and emergency pause mechanisms
Contract Architecture
Our smart contract architecture follows a modular design pattern that separates concerns and enables upgradability:
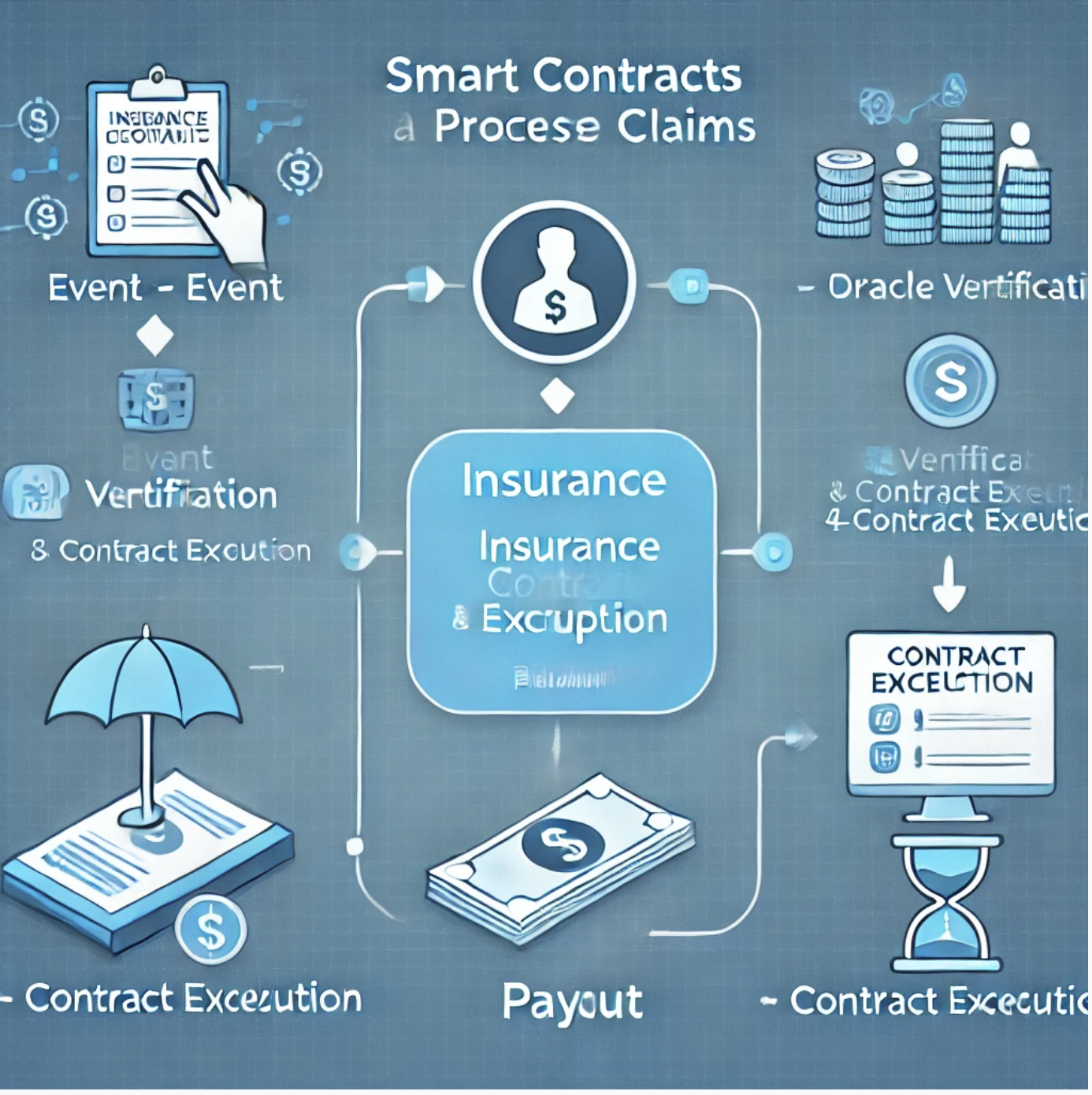
DefiLean's smart contract architecture
The main components of our architecture include:
- Registry Contract: Central registry that tracks all policies, products, and staking pools
- Product Templates: Standardized contracts for each insurance product type
- Policy Factory: Creates new policy instances based on product templates
- Staking Pool: Manages capital from stakers and distributes rewards
- Oracle System: Provides verified external data for claim processing
- Governance: Controls system parameters and upgrades
Insurance Registry
The Insurance Registry is the central hub of the DefiLean protocol. It maintains a comprehensive record of all insurance products, active policies, and staking pools.
Registry Functions
The Registry contract provides the following key functions:
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.0;
interface IInsuranceRegistry {
// Product management
function registerProduct(address productAddress, string memory productType) external;
function deactivateProduct(address productAddress) external;
function getProductList() external view returns (address[] memory);
function isProductActive(address product) external view returns (bool);
// Policy tracking
function registerPolicy(address policyAddress, address product) external;
function getPoliciesForUser(address user) external view returns (address[] memory);
function getPoliciesForProduct(address product) external view returns (address[] memory);
// Staking pool management
function registerStakingPool(address poolAddress, address product) external;
function getStakingPoolsForProduct(address product) external view returns (address[] memory);
// System parameters
function getProtocolFee() external view returns (uint256);
function getMinimumCoverageAmount() external view returns (uint256);
function getMaximumCoverageAmount() external view returns (uint256);
}
Registry Access Control
The Registry implements role-based access control to ensure that only authorized entities can make changes:
- Admin Role: Can add or remove products and set system parameters
- Product Role: Products can register policies that they create
- Factory Role: Policy Factory can register new policies
Upgradeability
The Registry contract uses a transparent proxy pattern for upgradeability, allowing us to fix bugs or add features while maintaining state and address continuity.
Policy Contracts
Policy contracts represent individual insurance agreements between users and the protocol. Each policy is a separate contract instance created from a template for its specific insurance type.
Policy Lifecycle
A policy contract goes through several states throughout its lifecycle:
-
1
Creation
Policy is created with specific parameters (e.g., flight number, coverage amount)
-
2
Activation
Policy becomes active when premium payment is received
-
3
Coverage Period
Policy provides coverage for the specified duration
-
4
Claim Processing
If a covered event occurs, oracle data triggers claim verification
-
5
Payout or Expiration
Policy pays out if claim is valid, or expires if no claim occurs
Policy Interface
All policy contracts implement a standard interface to ensure consistency:
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.0;
interface IPolicy {
enum PolicyStatus { Created, Active, Claimed, Expired, Cancelled }
function activate() external payable;
function processClaim(bytes calldata oracleData) external;
function getStatus() external view returns (PolicyStatus);
function getCoverageAmount() external view returns (uint256);
function getPremiumAmount() external view returns (uint256);
function getPolicyholder() external view returns (address);
function getStartTime() external view returns (uint256);
function getEndTime() external view returns (uint256);
function getProductType() external view returns (string memory);
function getParameters() external view returns (bytes memory);
}
Example Policy Contract
Here's a simplified flight delay policy implementation:
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.0;
import "./IPolicy.sol";
import "./OracleConsumer.sol";
contract FlightDelayPolicy is IPolicy, OracleConsumer {
// Policy details
address public policyholder;
uint256 public coverageAmount;
uint256 public premium;
uint256 public startTime;
uint256 public endTime;
PolicyStatus public status;
// Flight specific parameters
string public flightNumber;
uint256 public departureDate;
uint256 public delayThreshold; // minutes
// Events
event PolicyActivated(address indexed policyholder, uint256 premium);
event ClaimPaid(address indexed policyholder, uint256 amount);
event PolicyExpired();
constructor(
address _policyholder,
uint256 _coverageAmount,
uint256 _premium,
uint256 _startTime,
uint256 _endTime,
string memory _flightNumber,
uint256 _departureDate,
uint256 _delayThreshold
) {
policyholder = _policyholder;
coverageAmount = _coverageAmount;
premium = _premium;
startTime = _startTime;
endTime = _endTime;
flightNumber = _flightNumber;
departureDate = _departureDate;
delayThreshold = _delayThreshold;
status = PolicyStatus.Created;
}
}
function activate() external payable override {
require(status == PolicyStatus.Created, "Policy already activated");
require(msg.value == premium, "Incorrect premium amount");
status = PolicyStatus.Active;
emit PolicyActivated(policyholder, premium);
}
}
function processClaim(bytes calldata oracleData) external override onlyOracle {
require(status == PolicyStatus.Active, "Policy not active");
// Decode oracle data
(uint256 delayDuration, bool flightCancelled) = abi.decode(oracleData, (uint256, bool));
// Check if claim conditions are met
if (delayDuration >= delayThreshold || flightCancelled) {
status = PolicyStatus.Claimed;
// Transfer coverage amount to policyholder
(bool success, ) = policyholder.call{value: coverageAmount}("");
require(success, "Transfer failed");
emit ClaimPaid(policyholder, coverageAmount);
}
} else if (block.timestamp > endTime) {
status = PolicyStatus.Expired;
emit PolicyExpired();
}
}
}
}
// Implement remaining interface methods...
}
Staking Contracts
The staking system allows users to provide capital to back insurance policies and earn rewards in return. Staking contracts manage this process in a secure and transparent manner.
Staking Pool Functions
Staking pools implement the following key functions:
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.0;
interface IStakingPool {
// Staking operations
function stake(uint256 amount, uint256 lockPeriod) external;
function withdraw(uint256 amount) external;
function claimRewards() external;
// View functions
function getStakeAmount(address staker) external view returns (uint256);
function getAvailableRewards(address staker) external view returns (uint256);
function getLockEndTime(address staker) external view returns (uint256);
function getTotalStaked() external view returns (uint256);
function getAPY() external view returns (uint256);
// Pool information
function getPoolType() external view returns (string memory);
function getSupportedProducts() external view returns (address[] memory);
function getMinimumStake() external view returns (uint256);
function getMaximumStake() external view returns (uint256);
}
Risk and Reward Mechanism
The staking system carefully balances risk and reward:
- Premium Sharing: Stakers receive a portion of policy premiums
- Risk Exposure: Staked capital backs insurance payouts if claims occur
- Capital Efficiency: Staking pools are designed for optimal capital utilization
- Risk Tranches: Different risk levels with corresponding reward rates
Senior Pool
Lower risk, lower rewards, first loss protection
Mezzanine Pool
Balanced risk-reward profile, moderate exposure
Junior Pool
Higher risk, higher rewards, first to absorb losses
Diversified Pool
Spreads risk across multiple insurance products
Oracle Integration
Oracle networks provide the external data needed to verify insurance conditions and trigger claims. DefiLean uses a combination of decentralized oracle networks to ensure data reliability and prevent manipulation.
Oracle Data Sources
Different insurance products rely on different data sources:
Insurance Product | Data Sources | Update Frequency |
---|---|---|
Flight Delay | FlightStats API, Chainlink, Aviation authorities | Real-time |
Event Cancellation | Event APIs, News feeds, Venue data | Hourly |
Stablecoin Depeg | DEX price feeds, CEX price APIs, Chainlink | Every block |
Weather Impact | Weather APIs, IoT sensors, Meteorological data | Hourly |
Oracle Security Model
Our oracle integration includes several security measures:
- Multiple Sources: Data is aggregated from multiple independent sources
- Consensus Mechanism: Requires agreement from most data providers
- Staking: Oracle providers stake tokens as collateral against incorrect data
- Timelock: Critical data uses a timelock to prevent flash attacks
- Dispute Resolution: Process for challenging potentially incorrect data
Oracle Interface
Insurance contracts interact with oracles through a standardized interface:
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.0;
interface IOracleProvider {
function requestData(
bytes32 requestId,
string memory dataType,
bytes memory parameters
) external returns (bytes32);
function fulfillRequest(
bytes32 requestId,
bytes memory result
) external;
function cancelRequest(bytes32 requestId) external;
function getResult(bytes32 requestId) external view returns (
bool fulfilled,
bytes memory result
);
}